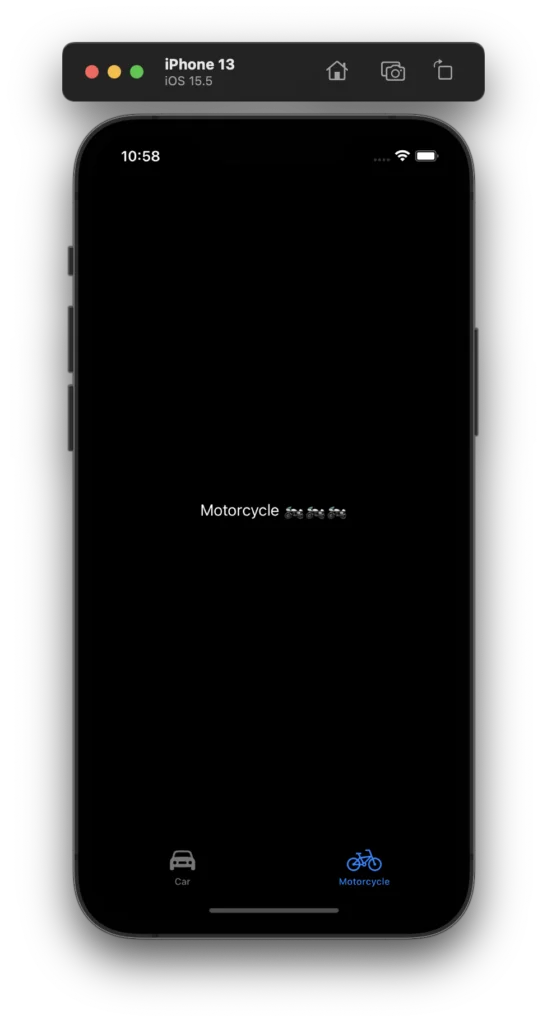
TabView
sample with tabs from enum
.
import SwiftUI
struct CustomTabBarView: View {
@State var selectedTab: TabBarItem
enum TabBarItem: String {
case car
case motorcycle
var title: String {
switch self {
case .car:
return "Car"
case .motorcycle:
return "Motorcycle"
}
}
var imageName: String {
switch self {
case .car:
return "car"
case .motorcycle:
return "bicycle"
}
}
}
var body: some View {
TabView(selection: $selectedTab) {
Text("Car πππ")
.tag(TabBarItem.car)
.tabItem {
Label(TabBarItem.car.title, systemImage: TabBarItem.car.imageName)
}
Text("Motorcycle πππ")
.tag(TabBarItem.motorcycle)
.tabItem {
Label(TabBarItem.motorcycle.title, systemImage: TabBarItem.motorcycle.imageName)
}
}
}
}
You can now use the TabView
in your navigation. This allows setting any tab as active tab.
import SwiftUI
struct ContentView: View {
var body: some View {
CustomTabBarView(selectedTab: .motorcycle)
}
}